What is DevSecOps?
Above all, DevSecOps is a cultural philosophy. It is a methodology that allows you to simultaneously achieve operational excellence and structural efficiency. DevSecOps provides major benefits to organizations:
- Increased speed for teams
- Faster and more frequent deliveries
- Application and deployment reliability
- Collaboration between stakeholders and improved scalability
- Security
What is Infrastructure as Code?
In most cases, the cloud makes it possible to do away with an infrastructure and the physical management thereof. Nevertheless, the infrastructure still exists. It simply adapts to the new methods and requirements of the teams. A migration to the cloud will therefore not make your Ops teams obsolete.
In fact, the rise of Infrastructure as Code (IaC) allows you to better serve all the players in your organization’s IT ecosystem. Often at the heart of DevOps Transformation, this approach is not just an added commodity, but a real necessity.
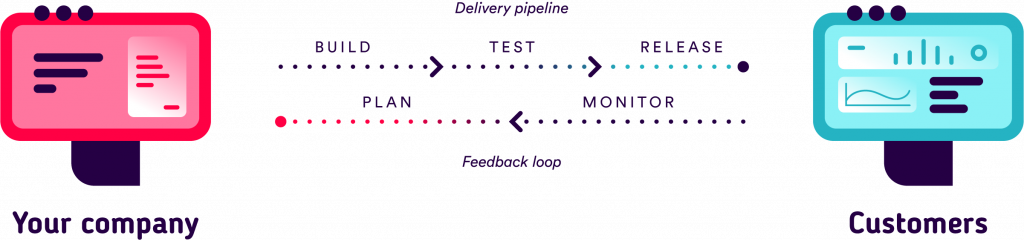
In addition to a reorganization to accommodate this paradigm shift, a number of tools and services (often associated with certain DevSecOps practices) are necessary for:
- Continuous integration (CI)
- Continuous delivery (CD)
- IaC
- Monitoring
- Collaboration
Tools, which are often considered simple commodities, are now vital to the implementation of DevSecOps in organizations. We therefore need to take some time to explore the various offers on the market, and understand how these products work together in your ecosystem.
How do AWS tools meet the requirements of DevSecOps?
Let’s look at the tools offered by AWS for creating a relevant and effective DevSecOps stack.
AWS tools for continuous integration (CI)
Continuous integration involves the automation of the build, test, and deliverable generation phases. It allows developers to be sure they have a sound foundation for each new project.
- CodeCommit is the first link in the chain, where the code is stored.
- CodeBuild implements the build, test, and deliverable generation phases. It can be launched at each commit in CodeCommit, for example.
- CodeArtifact is an artifact repository. It can be used to store CodeBuild results for projects such as a library. Let’s say the developers of another project need this library to be up to date to move forward with their project. A reference to the artifact repository will then be available in their dependency management tools.
- Elastic Container Registry (ECR) is a Docker image registry. It can be used in the same way as CodeArtifact, assuming CodeBuild has generated one or more Docker images, and that these images are useful for other projects or for the delivery phase.
AWS tools for continuous delivery (CD)
Continuous delivery involves the automation of the deployment phases, with the exception of production deployment (which is done manually).
- CodeDeploy makes it possible to deploy or update an application on AWS or on-premise servers.
- CodePipeline serves as the link between CI and CD. A pipeline defines a workflow that can start with the commit and extend all the way to the deployment:
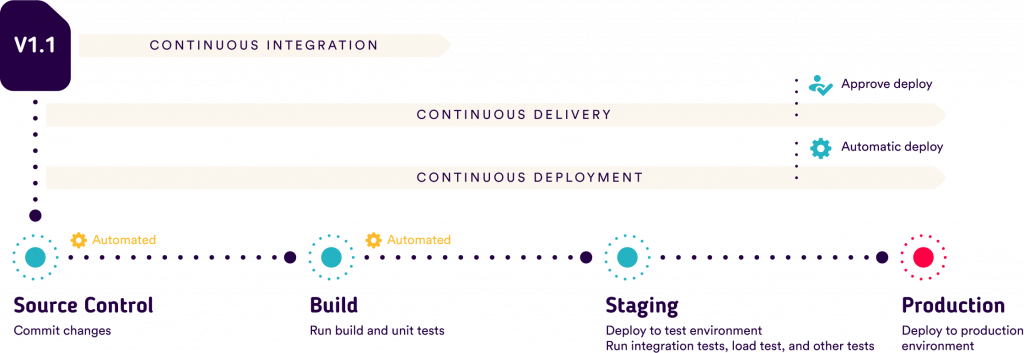
Which AWS tools can be used for Infrastructure as Code?
- CDK (Cloud Development Kit) can be used to set up the infrastructure (that will run your project) as code.
Compatible with JavaScript, TypeScript, Python, Java, C#, and Go (in Developer Preview), it transforms your favorite programming language into an AWS configuration managed by CloudFormation. - CloudFormation can be used directly to automate the instantiation of resources in AWS.
Let’s look at a simple example in TypeScript. We’ll create an AWS-managed secret that can only be read by a defined IAM role.
First of all, AWS provides us with a Stack interface to help us define our AWS infrastructure:
export class InfraStack extends Stack { constructor(scope: Construct, id: string, props?: StackProps) { super(scope, id, props); // definition of the infrastructure here } }
All the subsequent code blocks will be placed in “definition of the infrastructure here.”
Let’s create the IAM role:
const secretRole = new iam.Role(this, 'MyRole', { assumedBy: new iam.AnyPrincipal() });
This is stored in the secretRole variable, which can be used for the rest of the code.
“MyRole” is used to define the name that will be found in CloudFormation.
“assumedBy” lets you restrict who can assume the role. You may wish for the role to be assumed by a WebIdentityPrincipal like a federated web identity provided by Facebook, Google, Cognito, etc., a ServicePrincipal like Lambda or EC2, or a CanonicalUserPrincipal (an AWS user), etc.
Now that we have the role, let’s create the secret:
const websiteAccessPassword = new sm.Secret(this, "WebsiteAccessPassword", { generateSecretString: { secretStringTemplate: "{}", generateStringKey: "password" } });
First, we’ll initialize the secret “WebsiteAccessPassword” in CloudFormation. With this initialization, AWS generates a string that will correspond to the password contained in the secret.
“generateStringKey” generates the string in “password,” and the “password” key is found in the empty JSON object “{}” provided by “secretStringTemplate.”
In other words, when we retrieve the secret (for example, with the CLI command “aws secretsmanager get-secret-value”), it will give us a JSON object like this:
{ “password”: “leSecretGénéré” }
Finally, we must give our role permission to read our secret:
websiteAccessPassword.grantRead(secretRole);
Bonus:
We can add this role to any resource we created. For example, we may wish to access the secret from an EC2 instance that we’ve also created via CDK (or that we’ve referenced). Now you just have to give it the role “secretRole:”
const ec2Instance = new ec2.Instance(this, "ec2-instance", { “role”: secretRole, ... });
To finish with CDK, a single command suffices to transform our code into CloudFormation infrastructure: “cdk deploy”
Like all source codes, IaC can be managed with continuous integration and delivery. We can then deploy an entire infrastructure in the same way as we would with the programs that will run on this same infrastructure.
In this first article, we presented the AWS tools for the delivery, continuous deployment, and creation of Infrastructure as Code. These first bricks are the foundation of your AWS infrastructure. However, in order to adhere to DevSecOps methodology as closely as possible and obtain a consistent stack, additional bricks are required: in particular, monitoring and collaboration. These will be covered in subsequent articles. In the meantime, discover our AWS offer here.